Java: <...> (less than/greater than) syntax
The <...>
syntax allows you to write generic classes and methods that can handle multiple different types. This means, for instance, that you don't have to write one list class that can store integers, one list class that can store strings, and so on. Instead you can write one list class that's generic and can be used for any type.
ArrayList
does precisely this. It is parametrized on type E
…
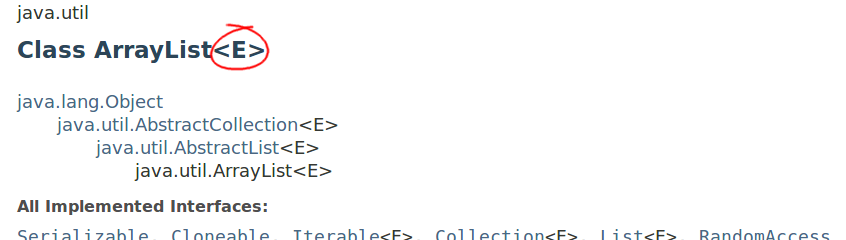
…which means that you can give it a type argument as follows:
ArrayList<String> strList;
In this case strList
will only contain objects of type String
and something like strList.add(5)
will fail to compile.
Looking at the documentation, we see that the ArrayList.get(int)
method returns something of type E
:
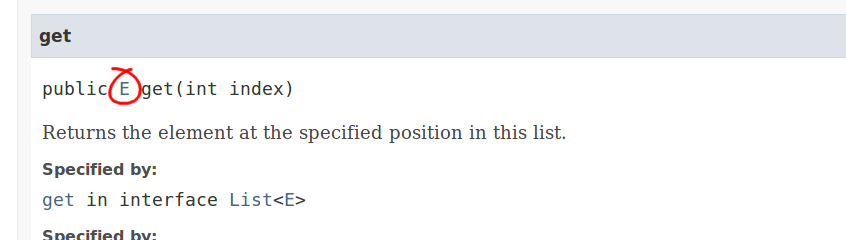
This means that strList.get()
will return a String
.
Diamond Syntax: new ArrayList<>()
If the type is left out, as below, the type is inferred:
List<String> l1 = new ArrayList<>();
List<String> l2 = new ArrayList<String>(); // Equivalent
Details
-
ArrayList
(without any type argument) is called the raw type and should not be used if it can be avoided. -
ArrayList<Object>
is not a super type ofArrayList<String>
. In technical terms: generic classes are invariant (as opposed to arrays that are covariant).